Testing and debugging strategies for a booking application are crucial for delivering a robust and reliable user experience. This exploration delves into the multifaceted world of software testing, encompassing unit, integration, system, and user acceptance testing. We will examine effective debugging techniques for common issues, explore performance and load testing for scalability, and address vital security considerations. Finally, we’ll discuss the implementation of a suitable test automation framework to ensure ongoing quality and efficiency.
The process involves meticulous planning and execution, from designing comprehensive test cases to leveraging automated tools for efficiency. Understanding different testing methodologies and their application to a booking system is paramount, allowing developers to identify and rectify defects before deployment. This comprehensive approach guarantees a high-quality application that meets user expectations and performs reliably under various conditions.
Unit Testing Strategies for Booking Application Functionality
Unit testing forms the bedrock of robust software development. For a booking application, where accuracy and reliability are paramount, a comprehensive unit testing strategy is crucial to ensure individual components function correctly before integration. This section details strategies for effectively unit testing core booking application functionalities.
Unit Test Cases for Core Booking Functions
A well-structured unit test suite covers various aspects of the booking process. The following table illustrates example test cases for date selection, user authentication, and payment processing. Remember that this is not an exhaustive list, and the specific tests will depend on the application’s features and complexity.
Test Case | Expected Result | Actual Result | Pass/Fail |
---|---|---|---|
Select a valid date range | Booking is allowed within the selected range. | Booking allowed. | Pass |
Select an invalid date range (past dates) | Error message indicating past dates are not allowed. | Error message displayed. | Pass |
Select a date with no availability | Error message indicating no availability on the selected date. | Error message displayed. | Pass |
Successful user login with valid credentials | User is redirected to the booking page. | User redirected. | Pass |
Failed user login with invalid credentials | Error message indicating incorrect username or password. | Error message displayed. | Pass |
Successful payment processing with valid card details | Payment is confirmed, and a booking confirmation is generated. | Payment confirmed, booking confirmed. | Pass |
Failed payment processing with invalid card details | Error message indicating payment failure. | Error message displayed. | Pass |
Mocking and Stubbing Dependent Services
Many booking application functions rely on external services, such as payment gateways or external APIs for availability checks. Directly testing these interactions in unit tests can be slow, unreliable, and prone to errors. Mocking and stubbing provide solutions. Mocking replaces a dependency with a controlled, simulated object, allowing for precise control over its behavior. Stubbing involves providing canned responses to method calls, simplifying testing and isolating the unit under test.
For example, when testing payment processing, a mock payment gateway can simulate successful or failed transactions without actually processing real payments. This ensures faster, more reliable, and repeatable tests.
Writing Effective Assertions
Assertions are crucial for verifying the correctness of test cases. They check whether the actual results match the expected results. Effective assertions must cover various data types and scenarios. For example, when testing date selection, assertions should check if the selected date is within the allowed range, and if the date format is correct. When testing user authentication, assertions should verify the user’s identity and access rights.
For payment processing, assertions should check the transaction status, amount, and other relevant details. Different assertion libraries provide methods for checking various data types (strings, numbers, dates, objects) and conditions (equality, inequality, membership, etc.). For instance, an assertion might check if a string is empty, if a number is within a certain range, or if a date is valid.
Choosing the appropriate assertion is vital for creating reliable and informative tests.
Integration Testing for Booking Application Modules
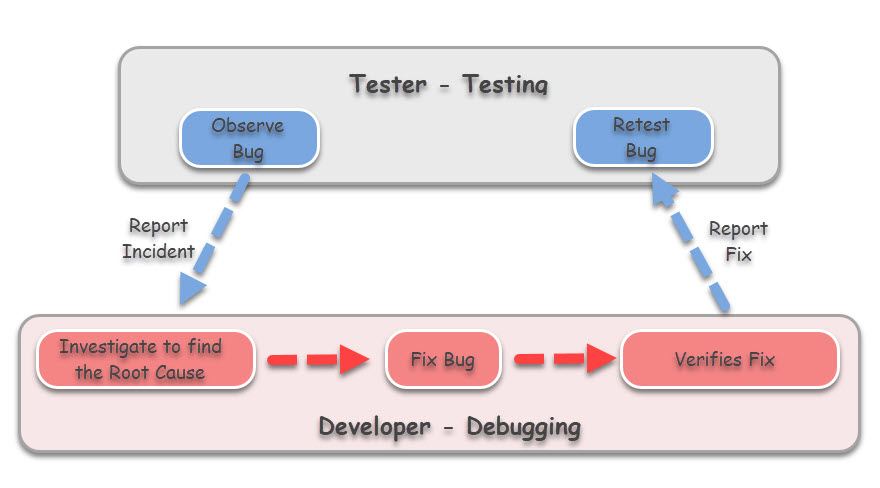
Source: toolsqa.com
Integration testing verifies the interaction between different modules of the booking application, ensuring they work together seamlessly. This is crucial for identifying issues that might not be apparent during unit testing, which focuses on individual components in isolation. Successful integration testing provides confidence that the system behaves as expected when different parts interact, ultimately leading to a more robust and reliable booking application.Integration testing for a booking application involves systematically combining and testing various modules, such as the booking engine, payment gateway, and user interface.
This process reveals flaws in communication and data exchange between these modules, preventing unexpected behavior in the live application. By simulating real-world scenarios, we can identify and resolve integration-level defects early in the development lifecycle.
Integration Testing Approaches: Top-Down vs. Bottom-Up
Top-down integration testing starts with the highest-level module and progressively integrates lower-level modules. This approach allows for early validation of the core functionality and helps identify high-level design flaws early on. Conversely, bottom-up integration testing begins with the lowest-level modules and gradually integrates them upwards. This approach can help identify problems in lower-level components before they impact higher-level modules.
The choice between these approaches often depends on the application’s architecture and the priorities of the testing effort. For example, in a booking application, a top-down approach might prioritize testing the user interface’s interaction with the booking engine first, while a bottom-up approach might focus on testing the payment gateway’s functionality before integrating it with the booking engine.
Example Integration Tests
Let’s consider a scenario where a user books a flight through the application. An integration test would cover the following interactions:* User Interface (UI) to Booking Engine: The test would simulate a user selecting a flight, entering passenger details, and submitting the booking request through the UI. This verifies that the UI correctly communicates the booking information to the booking engine.
Booking Engine to Payment Gateway
Upon receiving the booking request, the booking engine would initiate a payment request to the payment gateway. The integration test verifies that the correct information (amount, passenger details, etc.) is sent to the payment gateway and that the response is correctly handled by the booking engine.
Payment Gateway to Booking Engine
The payment gateway processes the payment and sends a confirmation or rejection to the booking engine. The integration test verifies that the booking engine handles both successful and failed payment responses appropriately, updating the booking status accordingly.
Booking Engine to UI
Finally, the booking engine updates the UI to reflect the booking status (confirmed, pending, failed). The integration test verifies that the UI correctly displays the updated status to the user.
Managing Test Data and Dependencies
Effective management of test data and dependencies is vital for successful integration testing. Poorly managed data can lead to inaccurate test results and make it difficult to reproduce issues.
Effective testing and debugging strategies for a booking application often involve simulating various user scenarios. This rigorous process ensures a seamless user experience, much like the meticulous design required for smart home automation for improved interior comfort and convenience , where reliability and responsiveness are paramount. Returning to the booking app, thorough testing minimizes glitches and improves overall functionality.
- Test Data Isolation: Use separate databases or test environments to isolate test data from production data. This prevents accidental modification of production data and ensures consistent test results.
- Data Setup and Teardown: Implement mechanisms to automatically set up and tear down test data before and after each test. This ensures that each test starts with a clean state and avoids dependencies between tests.
- Stubbing and Mocking: Use stubbing or mocking techniques to simulate external dependencies, such as the payment gateway, during integration tests. This isolates the tests from the actual payment gateway, allowing for more reliable and repeatable tests, especially during early development stages.
- Dependency Injection: Employ dependency injection to decouple modules and make it easier to swap real components with mock or stubbed versions during testing. This improves test flexibility and maintainability.
System Testing for End-to-End Booking Scenarios
System testing focuses on verifying the complete booking application, ensuring all integrated components work seamlessly together as expected. This phase simulates real-world user interactions to identify any flaws that might have been missed during unit and integration testing. It’s crucial for delivering a robust and reliable application to end-users.System testing involves executing a series of tests that cover the entire booking process, from initial search to final confirmation and beyond.
These tests validate the application’s functionality, performance, and security under realistic conditions. Thorough system testing minimizes the risk of unexpected issues arising after deployment.
Designing System Tests for Real-World User Flows
Effective system tests mimic how users actually interact with the application. This includes scenarios like booking creation (selecting dates, times, services, and adding passengers), modification (changing dates, adding extras, etc.), and cancellation (processing refunds and updating availability). Test cases should cover both successful and unsuccessful scenarios, including edge cases and boundary conditions. For example, a test case could verify that the system correctly handles a booking request for a date that falls outside the available range or that it properly manages concurrent booking requests to avoid overbooking.
Another test could involve verifying the system’s response to invalid input, such as incorrect date formats or missing passenger information. These tests help ensure the system behaves correctly under various circumstances.
System Test Plan
A comprehensive test plan is essential for successful system testing. This plan should clearly define the scope, objectives, and execution strategy.
- Scope: Define the specific features and functionalities to be tested. This might include booking creation, modification, cancellation, payment processing, user account management, and reporting features. It should also specify the different types of users (e.g., administrators, customers) and devices (e.g., desktop, mobile) to be considered.
- Objectives: State the goals of the system testing process. This could include verifying the functionality, performance, security, and usability of the application. Specific, measurable, achievable, relevant, and time-bound (SMART) objectives should be established.
- Test Environment: Describe the hardware and software configuration used for testing. This includes specifying the operating systems, browsers, databases, and other relevant components. A dedicated test environment that mirrors the production environment as closely as possible is crucial for accurate results.
- Test Cases: Detail the individual test cases to be executed. Each test case should have a unique identifier, a description of the test steps, expected results, and actual results. A traceability matrix linking test cases to requirements is also beneficial.
- Execution Strategy: Artikel the approach for executing the test cases. This might involve defining the test execution schedule, assigning responsibilities to team members, and specifying the tools and techniques to be used. This section could also detail how test results will be documented and analyzed.
- Risk Management: Identify potential risks and mitigation strategies. This could include issues related to test environment setup, test data management, and resource availability.
Handling Unexpected Errors and Exceptions
During system testing, unexpected errors and exceptions are inevitable. A robust error handling mechanism is crucial to prevent application crashes and data corruption. The system should gracefully handle these situations, providing informative error messages to the user and logging the details for debugging purposes. For instance, if a database connection fails, the system should display a user-friendly message indicating the problem and attempt to reconnect after a short delay.
Robust testing and debugging strategies are crucial for a successful booking application, ensuring a seamless user experience. For example, consider the complexities involved in managing user preferences, much like choosing the right layout for a smart home, which you can easily plan with the help of resources such as top smart home interior design software and apps for planning.
Returning to the booking app, thorough testing will uncover and resolve issues like payment processing errors or inaccurate availability displays before launch.
Detailed error logs should be generated to assist in identifying the root cause of the problem. These logs should include timestamps, error messages, and relevant stack traces. Furthermore, automated alerts could be implemented to notify the development team of critical errors. This proactive approach ensures rapid response to problems and minimizes downtime.
User Acceptance Testing (UAT) for Booking Application Validation
User Acceptance Testing (UAT) is the final stage of testing before a booking application is released to the public. It’s crucial for validating that the application meets the needs and expectations of its intended users, ensuring a positive user experience and minimizing post-release issues. This phase focuses on real-world scenarios and user workflows, providing valuable feedback to improve the application’s usability and functionality.UAT involves a systematic process of verifying that the application functions correctly from the perspective of end-users.
This goes beyond functional testing; it considers aspects such as user-friendliness, intuitive navigation, and overall satisfaction. The goal is to identify any remaining usability or functional flaws before the application is deployed.
Key Acceptance Criteria for the Booking Application
The acceptance criteria for the booking application are derived directly from the user requirements gathered during the initial phases of development. These criteria define the specific functionalities and performance levels that must be met for the application to be considered acceptable. They are typically documented in a formal acceptance test plan. Examples include successful booking completion, accurate pricing calculations, secure payment processing, clear cancellation policies, and effective customer support integration.
Failure to meet these criteria could lead to rejection of the application.
UAT Process Involving Diverse User Groups and Feedback Mechanisms
A robust UAT process involves engaging diverse user groups representing different demographics, technical skills, and booking behaviors. This ensures a comprehensive evaluation of the application’s usability and functionality across various user profiles. Feedback mechanisms should be multiple and accessible. These might include structured questionnaires, usability testing sessions with direct observation, online feedback forms, and user interviews. Regular communication and updates to participants are vital to maintain engagement and encourage detailed feedback.
For example, a diverse user group might include frequent travelers, occasional users, elderly users, users with disabilities, and users from different technological backgrounds.
Best Practices for Reporting and Managing UAT Defects
Effective defect reporting and management are essential for a successful UAT. A standardized defect tracking system should be used to document all identified issues. Each defect should include a clear description, steps to reproduce, expected behavior, actual behavior, severity level (e.g., critical, major, minor), and priority level (e.g., high, medium, low). A dedicated team should be responsible for analyzing, prioritizing, and resolving these defects.
Robust testing and debugging strategies are crucial for a successful booking application, ensuring a smooth user experience. This involves various approaches, from unit testing individual components to comprehensive end-to-end testing. Consider the complexity involved; it’s akin to the challenges of seamlessly integrating smart home technology into traditional interior styles, as discussed in this insightful article: integrating smart home technology into traditional interior styles.
Ultimately, thorough testing, much like a well-designed smart home system, guarantees a positive and functional outcome for the booking application.
Regular status reports should be provided to stakeholders, highlighting the progress of defect resolution and overall UAT progress. This ensures transparency and allows for timely intervention if necessary. Prioritizing defects based on their severity and impact on the user experience is critical to ensure that the most critical issues are addressed first. For instance, a critical defect might be a system crash during payment processing, while a minor defect might be a typographical error on a help page.
Debugging Techniques for Common Booking Application Issues
Effective debugging is crucial for maintaining a robust and reliable booking application. Identifying and resolving issues quickly minimizes downtime and improves user experience. This section details strategies for tackling common problems, focusing on practical techniques and tools.Debugging database errors, API failures, and UI glitches requires a systematic approach. Understanding the application’s architecture and the potential points of failure is the first step towards efficient troubleshooting.
The tools and techniques employed will depend on the specific nature of the issue.
Database Error Debugging
Database errors often stem from incorrect queries, connection problems, or data integrity issues. Thorough logging is essential for identifying the source of the problem. A well-structured logging system records relevant information such as timestamps, error messages, and potentially the SQL query that caused the error. This detailed information allows developers to quickly pinpoint the problematic area.
- Examine database logs: Most database systems maintain detailed logs of all activity. These logs provide valuable clues about failed queries, connection errors, or other database-related problems.
- Use a database debugger: Specialized database debuggers allow step-by-step execution of SQL queries, helping to identify the exact point of failure within complex queries.
- Check connection parameters: Verify that the database connection string is correctly configured, including the hostname, port, database name, username, and password. Incorrect credentials or network issues can lead to connection failures.
- Validate data integrity: Data integrity issues can lead to application errors. Regularly run database checks to identify and resolve inconsistencies in the data.
API Failure Debugging
API failures can result from network connectivity issues, incorrect API requests, or problems on the server-side. Again, logging plays a critical role, this time recording API request details, responses, and any error messages.
- Inspect API request and response: Use network monitoring tools (like browser developer tools or specialized network sniffers) to examine the details of API requests and responses. This allows verification of correct parameters, headers, and response status codes.
- Utilize API debugging tools: Many API platforms offer debugging tools that provide insights into the request and response cycle, including detailed error messages.
- Check server-side logs: Examine the server logs for errors or exceptions that occurred during API processing. This can reveal issues with server-side code or resource limitations.
- Simulate API calls: Use tools like Postman or curl to manually simulate API calls, helping to isolate whether the problem lies in the client-side code or the API itself.
UI Glitch Debugging
UI glitches often manifest as visual inconsistencies, unexpected behavior, or crashes. Browser developer tools are invaluable in this context, offering detailed information about the rendered HTML, CSS, and JavaScript.
- Inspect element: Use the “Inspect Element” functionality in your browser to examine the HTML, CSS, and JavaScript associated with the problematic UI element. This helps to identify styling conflicts, JavaScript errors, or other issues affecting the rendering.
- Use the browser’s JavaScript debugger: Set breakpoints in your JavaScript code to step through the execution and identify the source of errors or unexpected behavior.
- Check browser compatibility: Ensure that your UI is compatible with different browsers and devices. Inconsistencies across different browsers can lead to UI glitches.
- Test with different screen sizes: Test your UI on different screen sizes to ensure responsiveness and avoid layout issues.
Performance Bottleneck Isolation and Resolution
Performance bottlenecks can significantly impact user experience. Identifying and resolving these issues requires a combination of profiling tools and code analysis.
- Profiling tools: Use profiling tools to identify performance bottlenecks in your code. These tools measure execution times of different code sections, helping pinpoint slow parts of the application.
- Database query optimization: Inefficient database queries are a common source of performance problems. Optimize queries by using appropriate indexes, avoiding full table scans, and optimizing query structure.
- Caching strategies: Implement caching mechanisms to reduce database load and improve response times. Caching frequently accessed data can significantly improve performance.
- Code optimization: Optimize code for efficiency by reducing unnecessary computations, improving algorithm efficiency, and using appropriate data structures.
Performance and Load Testing for Scalability and Stability
Ensuring a booking application can handle a large number of concurrent users without performance degradation is crucial for its success. Performance and load testing are vital steps in achieving this scalability and maintaining system stability under pressure. These tests help identify bottlenecks and areas for improvement before the application is released to the public, preventing potential outages and negative user experiences.Performance testing evaluates the application’s response time and resource consumption under various conditions.
This involves simulating different user loads and monitoring key performance indicators (KPIs) such as response times, transaction throughput, and resource utilization (CPU, memory, network). Load testing, a subset of performance testing, focuses specifically on the application’s behavior under heavy loads, pushing it to its limits to determine its breaking point and identify potential stability issues. This information allows developers to proactively address performance bottlenecks and optimize the application for peak usage.
Performance Test Design and Execution
Designing effective performance tests requires a clear understanding of the application’s expected workload. This involves identifying critical user scenarios, such as making a booking, searching for availability, and managing user accounts. Test scenarios should simulate these scenarios with varying levels of concurrency to assess performance under different load conditions. Tools like JMeter or LoadRunner can be used to generate simulated user traffic and monitor performance metrics.
The tests should be designed to cover a range of load conditions, from low to extremely high, to identify the application’s breaking point and understand its behavior across the entire spectrum. For instance, a test might simulate 100 concurrent users making bookings simultaneously, then gradually increase the load to 500, 1000, and beyond to observe performance degradation.
Effective testing and debugging for a booking application often involves simulating various user scenarios. For instance, ensuring seamless integration with external services, such as verifying payment gateways, is crucial. This meticulous approach mirrors the detailed planning needed when designing a home’s lighting scheme, perhaps using smart home lighting solutions for enhancing interior ambiance , where careful consideration of color temperature and brightness is key.
Returning to the application, thorough testing ultimately ensures a reliable and user-friendly booking experience.
Performance Test Result Interpretation and Optimization
Analyzing performance test results involves examining key metrics such as response times, throughput, error rates, and resource utilization. Long response times indicate bottlenecks that need addressing. High error rates suggest instability under load. High CPU or memory utilization might point to inefficient code or inadequate hardware resources. Identifying these issues requires careful analysis of the test results, correlating performance metrics with specific application components or code sections.
For example, if the database query response time is consistently high during peak load, database optimization is necessary. Similarly, slow response times for specific API endpoints might indicate the need for code refactoring or caching strategies. The goal is to identify the root cause of performance issues and implement appropriate optimizations to improve the application’s responsiveness and stability.
Strategies for Scaling the Application
Scaling a booking application to handle peak loads often involves a combination of techniques. Vertical scaling, increasing the resources of existing servers (more RAM, faster processors), is a simpler approach but has limitations. Horizontal scaling, adding more servers to distribute the load, is more scalable and robust. Load balancing distributes incoming requests across multiple servers, preventing any single server from becoming overloaded.
Caching frequently accessed data reduces database load and improves response times. Database optimization, such as indexing and query tuning, can significantly improve database performance. Content Delivery Networks (CDNs) can distribute static content (images, CSS, JavaScript) closer to users, reducing latency. Employing a microservices architecture allows independent scaling of individual application components, offering greater flexibility and resilience.
For example, during a holiday booking rush, the booking service microservice could be scaled horizontally to handle the increased demand, while other microservices remain at their normal capacity.
Security Testing for Booking Application Vulnerabilities
Protecting user data and maintaining the integrity of a booking application is paramount. Security testing plays a crucial role in identifying and mitigating potential vulnerabilities before they can be exploited. This section details common vulnerabilities, testing techniques, and best practices for securing a booking application.Security testing aims to proactively uncover weaknesses that could allow unauthorized access, data breaches, or system disruptions.
This involves a multifaceted approach, combining automated tools with manual testing to identify a wide range of vulnerabilities.
Potential Security Vulnerabilities
A booking application handles sensitive information like user credentials, payment details, and personal data, making it a prime target for malicious attacks. Common vulnerabilities include SQL injection, where malicious code is injected into database queries to manipulate data; cross-site scripting (XSS), allowing attackers to inject client-side scripts into web pages viewed by other users; and cross-site request forgery (CSRF), tricking users into performing unwanted actions.
Other potential vulnerabilities include insecure session management, insecure data storage, and lack of input validation. Failing to address these vulnerabilities could lead to significant financial and reputational damage.
Thorough testing of a booking application involves various strategies, from unit tests to integration tests, ensuring seamless functionality. Understanding user flows is crucial, much like considering the user experience when planning a smart home, as detailed in this guide on best practices for smart home interior design and installation. Similarly, effective debugging in the booking app requires a systematic approach, identifying and resolving issues before release to prevent user frustration.
Security Testing Techniques
Penetration testing simulates real-world attacks to identify vulnerabilities. Ethical hackers attempt to exploit weaknesses in the application’s security controls, providing valuable insights into potential attack vectors. Vulnerability scanning uses automated tools to identify known security flaws by analyzing the application’s code and configuration. Static analysis examines the code without executing it, while dynamic analysis involves testing the application in a runtime environment.
Regular security audits, code reviews, and security awareness training for developers are also critical components of a comprehensive security testing strategy.
Security Best Practices Checklist
Implementing robust security measures throughout the development lifecycle is crucial. This checklist Artikels key best practices:
- Input Validation: Sanitize all user inputs to prevent injection attacks (SQL injection, XSS).
- Secure Authentication and Authorization: Implement strong password policies, multi-factor authentication, and role-based access control.
- Secure Data Storage: Encrypt sensitive data both in transit and at rest. Use appropriate database security measures.
- Regular Security Updates: Keep all software components and libraries up-to-date with the latest security patches.
- Secure Session Management: Use secure session IDs and implement proper session timeouts.
- Output Encoding: Encode all output to prevent XSS vulnerabilities.
- Regular Security Testing: Conduct penetration testing and vulnerability scanning regularly to identify and address vulnerabilities proactively.
- Secure Coding Practices: Adhere to secure coding guidelines and best practices to minimize vulnerabilities.
- Error Handling: Implement robust error handling to prevent information leakage.
- Regular Security Audits: Conduct regular security audits to assess the overall security posture of the application.
Following these best practices significantly reduces the risk of security breaches and ensures the application’s long-term security and stability. For example, a well-known online retailer suffered a significant data breach due to a failure to properly sanitize user inputs, resulting in a SQL injection vulnerability that exposed customer data. This highlights the importance of diligent security testing and the implementation of robust security measures.
Test Automation Framework Selection and Implementation: Testing And Debugging Strategies For A Booking Application
Automating tests for a booking application significantly improves efficiency and reduces the risk of human error. Choosing the right automation framework is crucial for the success of this endeavor. This section will compare popular frameworks and detail the process of implementing an automated testing framework within a CI/CD pipeline.
Selecting an appropriate test automation framework depends heavily on the application’s architecture (web, mobile, desktop), testing needs, team expertise, and budget. A well-designed framework should be maintainable, scalable, and easily integrated into the CI/CD pipeline.
Comparison of Test Automation Frameworks
Several frameworks excel in different areas. The following table compares three popular choices: Selenium, Cypress, and Appium.
Feature | Selenium | Cypress | Appium |
---|---|---|---|
Programming Languages | Multiple (Java, Python, C#, JavaScript, etc.) | JavaScript | Multiple (Java, Python, JavaScript, Ruby, etc.) |
Browser Support | Wide range of browsers | Chrome, Firefox, Edge (with limitations on others) | Limited browser support directly; relies on underlying drivers |
Testing Type | Web, mobile (with Appium integration) | Primarily web | Mobile (native, hybrid, and mobile web) |
Ease of Use | Steeper learning curve | Relatively easier to learn and use | Moderate learning curve, requires understanding of mobile app testing concepts |
Debugging | Can be challenging, requires good understanding of browser developer tools | Excellent debugging capabilities, built-in developer tools | Debugging can be complex, requires familiarity with mobile device debugging tools |
Speed | Can be slower due to WebDriver overhead | Faster due to direct browser interaction | Speed varies depending on the mobile platform and app complexity |
Community Support | Large and active community | Growing and active community | Active community, though potentially smaller than Selenium’s |
Designing and Implementing an Automated Testing Framework
Designing and implementing an automated testing framework for the booking application involves several key steps. A well-structured framework ensures maintainability, reusability, and scalability.
The process typically includes:
- Framework Selection: Based on the comparison above and project requirements, choose a suitable framework (e.g., Selenium for web testing).
- Test Environment Setup: Configure the testing environment, including browsers, drivers, and necessary libraries.
- Test Case Design: Create clear, concise, and well-defined test cases covering critical booking application functionalities.
- Test Script Development: Write automated test scripts using the chosen framework, following best practices for code readability and maintainability.
- Test Data Management: Implement a strategy for managing test data, ensuring data consistency and preventing data corruption.
- Reporting and Logging: Integrate reporting mechanisms to track test execution results and generate comprehensive reports.
- Continuous Integration: Integrate the automated tests into a CI/CD pipeline to automate the testing process.
Integrating Automated Tests into the CI/CD Pipeline, Testing and debugging strategies for a booking application
Integrating automated tests into the CI/CD pipeline ensures that tests are executed automatically with every code change, providing immediate feedback on the quality of the application.
Rigorous testing and debugging are crucial for a successful booking application, ensuring seamless user experience and preventing costly errors. This meticulous approach mirrors the planning needed for achieving a sustainable and smart home interior design , where careful consideration of materials and functionality is paramount. Similarly, in application development, comprehensive testing, from unit tests to integration tests, is essential to guarantee a robust and user-friendly final product.
This integration typically involves:
- CI/CD Tool Selection: Choose a CI/CD tool (e.g., Jenkins, GitLab CI, Azure DevOps).
- Pipeline Configuration: Configure the CI/CD pipeline to trigger automated tests upon code commits or merges.
- Test Execution: Integrate the test scripts into the pipeline to execute them automatically.
- Results Analysis: Configure the pipeline to analyze test results, identify failures, and report them appropriately.
- Deployment Trigger: Set up the pipeline to trigger deployment only if the automated tests pass successfully.
Final Wrap-Up
In conclusion, implementing a robust testing and debugging strategy is paramount for the success of any booking application. By combining rigorous testing methodologies, effective debugging techniques, and a well-structured automation framework, developers can significantly improve the application’s quality, reliability, and security. Proactive identification and resolution of defects minimize post-release issues, ensuring a positive user experience and fostering customer satisfaction.
Continuous improvement and adaptation of testing strategies in response to evolving user needs and technological advancements remain key to long-term success.
Clarifying Questions
What are the common challenges in testing a booking application?
Common challenges include handling concurrent bookings, managing complex data interactions with external services (payment gateways, etc.), ensuring accurate data synchronization, and testing various user scenarios and edge cases.
How can I ensure my tests cover all possible scenarios?
Employ risk-based testing, prioritizing critical functionalities and user flows. Use test case design techniques like equivalence partitioning and boundary value analysis to effectively cover a wide range of inputs and scenarios.
What are some good practices for managing test data?
Use test data management tools, create realistic yet controlled test environments, and employ data masking techniques to protect sensitive information while maintaining data integrity.
How can I effectively integrate automated tests into a CI/CD pipeline?
Select a suitable CI/CD tool (e.g., Jenkins, GitLab CI), configure triggers for automated test execution upon code commits, and integrate reporting mechanisms to monitor test results and identify failures.
How do I choose the right test automation framework?
Consider factors such as application type (web, mobile), programming language, team expertise, and the framework’s capabilities and community support. Evaluate frameworks like Selenium, Cypress, or Appium based on your specific needs.
- AGC Glass A Global Leader in Glass Manufacturing - June 2, 2025
- Voice-controlled home Smart living simplified - May 6, 2025
- Smart Thermostat House Your Eco-Friendly Home - May 6, 2025