Implementing real-time availability updates in a booking system is crucial for providing a seamless and user-friendly experience. This enhancement transforms the often frustrating experience of checking availability into a smooth, instantaneous process. This document delves into the architectural considerations, data management strategies, user interface design, and testing procedures necessary to successfully implement this vital feature. We’ll explore various technologies and approaches, weighing their advantages and disadvantages to help you choose the best solution for your specific needs.
The goal is to build a robust, scalable, and reliable system that ensures accurate and consistent availability information for all users.
The process involves carefully selecting appropriate technologies, from message queues and databases to UI frameworks and testing methodologies. Careful consideration of concurrency, data consistency, and error handling are paramount. Ultimately, the successful implementation of real-time updates hinges on a holistic approach that encompasses all aspects of system design and development, from the backend architecture to the user-facing interface.
Technological Considerations for Real-Time Updates: Implementing Real-time Availability Updates In A Booking System
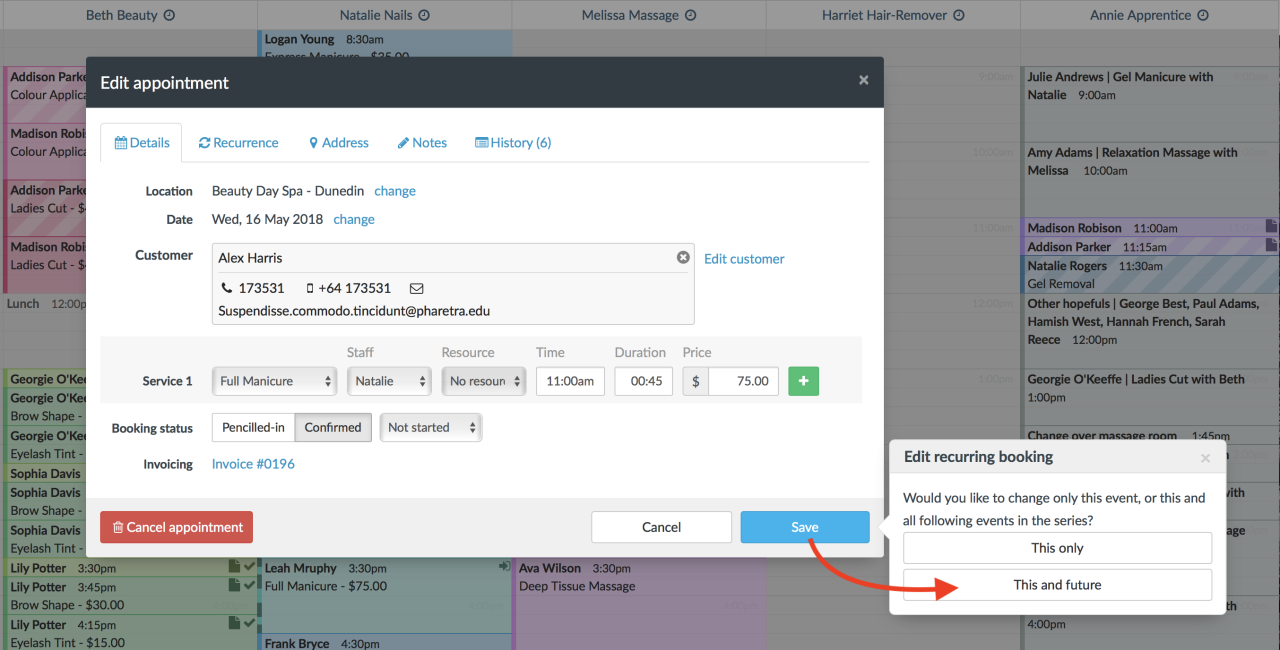
Source: cloudfront.net
Implementing real-time availability updates in a booking system requires careful consideration of various technological aspects to ensure efficiency, scalability, and responsiveness. The choice of architecture, messaging system, database, and communication protocol significantly impacts the system’s performance and user experience.
Architectural Patterns for Real-Time Updates
Several architectural patterns can effectively handle real-time data updates. The optimal choice depends on factors such as the expected volume of updates, latency requirements, and the complexity of the system.
Architecture | Scalability | Latency | Complexity |
---|---|---|---|
Event-Driven Architecture | High; easily scales horizontally by adding more event processors. | Low; updates are propagated asynchronously. | Moderate; requires careful design of event handling and message queues. |
Microservices Architecture | High; individual services can be scaled independently. | Can be low to moderate; depends on inter-service communication. | High; increased complexity in managing multiple services and their interactions. |
Publish-Subscribe Architecture | High; publishers and subscribers can be scaled independently. | Low; updates are broadcast to interested subscribers. | Moderate; requires a robust message broker and efficient topic management. |
Centralized Database with Polling | Low; scaling requires significant database upgrades. | High; clients need to periodically poll the database for updates. | Low; simpler to implement initially. |
The Role of Message Queues
Message queues like RabbitMQ and Kafka play a crucial role in ensuring efficient delivery of availability updates. They act as intermediaries, decoupling the system components responsible for generating updates from those consuming them. This improves reliability, scalability, and fault tolerance. For example, when a booking is made, a message indicating the updated availability is published to the queue. Consumers (e.g., other microservices, the frontend) subscribe to the queue and process the message accordingly.A simple example using RabbitMQ in Python could involve using the `pika` library to publish and consume messages.
The publisher would send a JSON message containing the updated availability details, and consumers would receive and process these messages to update their respective data stores and UI.
Database Technologies for Real-Time Updates
The choice of database technology significantly impacts the efficiency of real-time updates.
- Relational Databases (e.g., PostgreSQL, MySQL):
- Advantages: ACID properties ensure data consistency, mature ecosystem with robust tooling.
- Disadvantages: Can struggle with high write throughput and horizontal scaling; real-time updates may require complex queries and optimizations.
- NoSQL Databases (e.g., MongoDB, Cassandra):
- Advantages: Excellent scalability and performance for high write throughput; flexible schema adapts to evolving data needs.
- Disadvantages: May lack ACID properties; data consistency requires careful design and implementation; potentially less mature ecosystem than relational databases.
Implementing WebSockets or Server-Sent Events
WebSockets and Server-Sent Events (SSE) are technologies that enable pushing real-time updates to clients without the client needing to constantly poll the server. WebSockets provide a full-duplex communication channel, while SSE offers a unidirectional stream from server to client.A basic WebSocket handshake using JavaScript might look like this:“`javascriptconst socket = new WebSocket(‘ws://localhost:8080’);socket.onopen = function(event) console.log(‘WebSocket connection opened:’, event);;socket.onmessage = function(event) console.log(‘Received message:’, event.data);;socket.onclose = function(event) console.log(‘WebSocket connection closed:’, event);;“`This code snippet establishes a WebSocket connection, handles the connection events, and logs received messages.
The server-side implementation would involve handling the WebSocket connection and sending updates to connected clients.
Data Management and Synchronization Strategies
Implementing real-time availability updates in a booking system requires a robust strategy for managing concurrent access to booking data and ensuring data consistency across all clients. This involves careful consideration of locking mechanisms, conflict resolution, and efficient caching techniques. Failure to address these aspects can lead to data corruption, inaccurate availability information, and a poor user experience.
Concurrent Access Control and Race Conditions
Preventing race conditions, where multiple simultaneous updates lead to inconsistent data, is crucial. We can achieve this using either optimistic or pessimistic locking mechanisms. Pessimistic locking assumes conflicts are likely and locks the booking data before any modification. This prevents simultaneous access, ensuring data integrity but potentially impacting performance due to blocking. Optimistic locking, conversely, assumes conflicts are infrequent.
It allows concurrent access and only checks for conflicts when a user attempts to save changes. If a conflict is detected (e.g., another user modified the data in the meantime), the update is rejected, and the user is notified. The choice between these mechanisms depends on the expected concurrency level and the acceptable trade-off between performance and data integrity.
For high-concurrency systems, optimistic locking, coupled with efficient conflict resolution strategies, often provides a better balance.
Data Consistency and Update Process
Maintaining data consistency across all clients is paramount. This is achieved through a combination of techniques. A central database acts as the single source of truth. Upon a booking request, the system first checks availability in the database. If available, the system updates the database and simultaneously notifies all clients of the change via a real-time communication mechanism, such as WebSockets.
This ensures that all clients immediately reflect the updated availability. The following flowchart illustrates this process:[Flowchart Description: The flowchart begins with a “Booking Request” box. An arrow points to a “Check Availability (Database)” box. If available, an arrow leads to “Update Database” and “Notify Clients (WebSockets)”. If unavailable, an arrow leads to “Reject Booking”.
All boxes are connected by arrows indicating the flow of the process. The use of WebSockets ensures immediate updates are pushed to all connected clients, eliminating the need for polling.]
Implementing real-time availability updates in a booking system requires careful consideration of data management and user experience. This mirrors the challenges faced when designing smart homes, as highlighted in this article on balancing technology and aesthetics in smart home interior design ; both necessitate a seamless integration of functionality without compromising visual appeal. Ultimately, success in either endeavor hinges on prioritizing user satisfaction through intuitive and efficient design.
Conflict Resolution
When multiple users attempt to book the same resource simultaneously, a conflict arises. To resolve this, a first-come, first-served approach, enforced by the database’s locking mechanism, is typically used. In the case of optimistic locking, a conflict resolution algorithm is necessary. This algorithm could involve:
- Rejecting subsequent booking requests for the already-booked resource.
- Implementing a queuing system to handle pending booking requests in a fair manner.
- Notifying users of the conflict and allowing them to choose an alternative time slot.
The following pseudocode illustrates a simple conflict resolution algorithm using optimistic locking:“`function bookResource(resourceId, userId, bookingTime): // Optimistically read the resource availability resource = getResource(resourceId) if resource.isAvailable(bookingTime): // Attempt to update the resource availability success = updateResourceAvailability(resourceId, bookingTime, userId) if success: // Booking successful return “Booking confirmed” else: // Conflict detected return “Booking failed.
Resource already booked.” else: // Resource not available return “Booking failed. Resource unavailable at that time.”“`
Caching Strategies
Caching booking data significantly reduces database load and improves response times. However, different caching strategies have trade-offs. A simple approach is to cache the entire availability data for a specific period. This is efficient for read-heavy systems but requires frequent updates to maintain consistency. More sophisticated techniques include using cache invalidation mechanisms (e.g., removing cached data when an update occurs) or employing a distributed cache with data replication to handle high traffic.
The choice of caching strategy depends on the system’s scale, traffic patterns, and the acceptable level of data staleness. For instance, a system with high read-to-write ratios might benefit from aggressive caching, while a system with frequent updates might require a more dynamic caching approach.
Implementing real-time availability updates in a booking system requires careful consideration of data synchronization and user experience. This is analogous to the seamless integration needed for smart home security systems that complement interior aesthetics , where responsiveness and visual harmony are key. Ultimately, both systems benefit from a well-designed architecture ensuring instant and accurate information for the user.
User Interface and Experience Design
Designing a user-friendly interface for a booking system with real-time availability updates is crucial for a positive user experience. Clear communication of availability, coupled with graceful handling of potential delays or conflicts, is key to minimizing frustration and maximizing user satisfaction. The following sections detail the design considerations for achieving this.
Real-Time Availability Display
Effective visual cues are essential for conveying real-time availability. A color-coded calendar system, for instance, could use green for available slots, red for booked slots, and yellow for slots with limited availability. Hovering over a specific time slot could then reveal more detailed information, such as the number of remaining spots or any relevant restrictions. Additionally, a clear and concise legend explaining the color-coding should be readily visible on the page.
Real-time updates should be indicated by subtle animations, such as a brief flash of the updated slot’s color or a small “Updated” tag appearing next to it, ensuring the user is aware of the dynamic nature of the information.
Implementing real-time availability updates in a booking system is crucial for user experience. Think of the seamless integration needed; it’s akin to the smooth operation of a smart home, much like the elegant functionality showcased in this example of modern smart home interior design with minimalist furniture. Just as a smart home instantly reflects changes, a booking system should instantly update availability, preventing double-bookings and ensuring efficiency.
Wireframe Integration of Real-Time Updates
A typical booking system might include a calendar view, a list view of available times, and a booking confirmation page. The wireframe would integrate real-time availability across these sections. The calendar view would show color-coded availability in a grid format, updating dynamically as bookings are made or canceled. The list view would similarly reflect real-time changes, with available times highlighted and unavailable times grayed out or clearly marked as such.
The booking confirmation page would, of course, display the final confirmed booking details, including date, time, and any relevant information, thus reflecting the updated availability after the booking is successfully completed.
Handling Delays and Unavailable Updates
Situations where updates are delayed or unavailable require careful consideration. A prominent loading indicator, such as a spinning wheel or progress bar, should be displayed while the system fetches the latest availability information. If an update is significantly delayed, a user-friendly message such as “We’re experiencing a slight delay in updating availability. Please try again in a few moments.” should be shown.
In cases of complete unavailability, a clear error message, for example, “We are currently unable to retrieve availability information. Please try again later or contact our support team,” should be presented, accompanied by contact information for assistance.
Minimizing Confusion from Rapidly Changing Availability
Rapidly changing availability can be confusing. To mitigate this, the system should prioritize displaying the most up-to-date information clearly. Any changes should be visually distinct to avoid ambiguity. For instance, if a slot changes from available to booked, the change should be clearly indicated with an animation or a visual cue. If a booking conflict arises, a clear and concise message explaining the conflict should be displayed, perhaps offering alternative times or suggesting contacting customer support.
For example, a message could say: “Unfortunately, this time slot is no longer available. Would you like to see other available times or speak to someone?” This approach ensures transparency and empowers users to make informed decisions.
Testing and Deployment Strategies
Implementing real-time availability updates requires a robust testing and deployment strategy to ensure a seamless transition to a production environment and maintain system reliability. This section details the processes involved, from comprehensive testing to monitoring and handling potential failures.
Testing Strategy, Implementing real-time availability updates in a booking system
A multi-faceted testing approach is crucial for validating the accuracy and reliability of real-time availability updates. This involves a combination of unit, integration, system, and performance testing. Unit testing focuses on individual components, ensuring each function operates correctly in isolation. Integration testing verifies the interaction between different components, while system testing assesses the entire system’s functionality as a whole.
Finally, performance testing evaluates the system’s responsiveness and stability under various load conditions. These tests will help identify and resolve issues before deployment.
Unit Testing Objectives
Unit tests will focus on verifying the accuracy of individual functions responsible for fetching and updating availability data, such as database interactions and calculations. These tests will ensure that each component functions correctly and independently. Example unit tests could focus on verifying the correct calculation of available slots based on existing bookings or confirming the accurate retrieval of data from the database.
Integration Testing Objectives
Integration testing will cover the interactions between different modules, for example, the interaction between the availability update module and the booking module. Tests will verify that data is correctly passed between modules and that the overall system functions as designed. A test case might involve booking a slot and then verifying that the availability is correctly updated across all relevant systems.
Implementing real-time availability updates in a booking system requires a robust, responsive architecture. This is analogous to the seamless integration needed when, for example, you’re integrating smart home technology into traditional interior styles , ensuring all devices work harmoniously. Similarly, a booking system needs immediate, accurate feedback to avoid double-bookings and maintain user satisfaction.
System Testing Objectives
System testing will encompass end-to-end testing of the entire system, simulating real-world scenarios. This involves testing various user workflows and edge cases to identify any potential issues. For example, this could involve simulating a high volume of concurrent bookings to ensure the system remains responsive and accurate.
Implementing real-time availability updates in a booking system is crucial for user experience; instantaneous feedback prevents double-bookings and ensures a smooth process. This kind of responsiveness mirrors the seamless integration you’d expect in a well-designed smart home, like those detailed in this helpful guide on creating a luxurious smart home interior on a budget. Ultimately, both require careful planning and efficient data management to deliver a superior experience.
Performance Testing Objectives
Performance testing will focus on evaluating the system’s response time, scalability, and stability under different load conditions. This involves simulating various user loads to identify potential bottlenecks and ensure the system can handle expected traffic. Metrics like response time, throughput, and resource utilization will be closely monitored. For instance, a load test could simulate 100 concurrent users attempting to book slots simultaneously to gauge system performance.
Deployment Process
Deploying the updated system to a production environment requires a phased approach to minimize downtime and ensure a smooth transition. A common strategy involves using a blue-green deployment, where the new system runs in parallel with the existing system before switching over.
Implementing real-time availability updates in a booking system is crucial for a seamless user experience. This level of responsiveness mirrors the immediate feedback found in many helpful applications, such as those for interior design; for example, check out the top smart home interior design software and apps for planning to see how intuitive design can enhance user interaction.
Ultimately, this instant feedback translates directly to improved efficiency and customer satisfaction in your booking system.
Step-by-Step Deployment Guide
- Preparation: Thoroughly test the updated system in a staging environment mirroring the production environment.
- Deployment to Staging: Deploy the updated system to a staging environment identical to the production environment for final validation testing.
- Blue-Green Deployment: Deploy the updated system to a separate production environment (green). Simultaneously run both the old (blue) and new (green) systems.
- Traffic Switching: Gradually shift traffic from the old system (blue) to the new system (green) using a load balancer. Monitor performance closely during this transition.
- Validation: Verify the new system’s functionality and performance in the production environment. Conduct thorough testing to ensure everything works as expected.
- Decommissioning: Once the new system is fully validated, decommission the old system (blue).
Performance Monitoring
Continuous monitoring of the real-time update system in the production environment is crucial for identifying potential bottlenecks and ensuring high availability. Key performance indicators (KPIs) include response time, error rates, and resource utilization.
Monitoring Metrics
- Response Time: The time taken to update availability information. Slow response times can indicate bottlenecks.
- Error Rate: The percentage of failed update attempts. High error rates signal problems that need immediate attention.
- Resource Utilization: CPU, memory, and network usage. High utilization can lead to performance degradation.
- Availability: The percentage of time the system is operational. Downtime should be minimized.
Error Handling and Recovery
Unexpected errors or failures require robust mechanisms for automatic recovery and fallback solutions. A well-defined strategy will minimize disruption and ensure system resilience.
Failure Handling Decision Tree
A decision tree will guide the response to different failure types. For instance, a database connection failure might trigger a failover to a secondary database, while a software error might trigger an automatic restart. The severity of the error and the impact on users will determine the appropriate response.
A simple example: If database connection fails, attempt reconnection. If reconnection fails after three attempts, switch to backup database and send an alert. If the backup database also fails, trigger an alert and initiate manual intervention.
Final Summary
Successfully implementing real-time availability updates in a booking system significantly enhances the user experience, leading to increased customer satisfaction and potentially higher conversion rates. By carefully considering the architectural patterns, data management strategies, user interface design, and rigorous testing, developers can create a system that is both efficient and reliable. The careful selection of technologies and the implementation of robust error-handling mechanisms are vital for ensuring a smooth and seamless experience for users, even under high load or unexpected events.
This approach ultimately contributes to a more efficient and enjoyable booking process for all.
Frequently Asked Questions
What are the potential security risks associated with real-time updates, and how can they be mitigated?
Real-time updates introduce potential security vulnerabilities, such as unauthorized access or modification of booking data. Mitigation strategies include robust authentication and authorization mechanisms, input validation, secure communication protocols (HTTPS), and regular security audits.
How can I handle situations where the real-time update fails or is significantly delayed?
Implement graceful degradation mechanisms, such as displaying a cached version of availability with a clear indication that the data might be slightly outdated. Provide informative error messages to users and consider implementing fallback mechanisms to access data from a secondary source.
What are the best practices for monitoring the performance of the real-time update system?
Establish key performance indicators (KPIs) such as update latency, success rate, error rate, and resource utilization. Use monitoring tools to track these KPIs and set up alerts for potential issues. Regularly review and adjust your monitoring strategy based on system performance and evolving needs.
How do I choose the right message queue for my booking system?
The choice depends on factors such as message volume, throughput requirements, and message ordering needs. RabbitMQ is suitable for smaller systems, while Kafka excels in high-throughput environments. Consider factors like scalability, reliability, and ease of integration with your existing infrastructure.
- Different Styles of Outdoor Lighting for Various Home Designs - February 21, 2025
- Finding the perfect balance between security and ambiance with outdoor lighting - February 21, 2025
- Home Security Automation System Compatibility with Smart Assistants - February 18, 2025