Building a scalable booking application for high traffic presents unique challenges. Successfully navigating these requires a robust architecture, efficient handling of concurrent requests, and sophisticated caching strategies. This exploration delves into the crucial aspects of designing, developing, and deploying a booking system capable of withstanding substantial and fluctuating user loads, ensuring seamless performance even during peak demand. We’ll examine architectural choices, concurrency management, caching techniques, API optimization, security measures, and deployment strategies to build a resilient and scalable solution.
The complexities involved extend beyond simply choosing the right technologies. Strategic planning is paramount, encompassing considerations such as database selection (balancing scalability and cost), load balancing techniques, API design for optimal performance and maintainability, and a comprehensive testing and monitoring regime to identify and address potential bottlenecks proactively. This detailed approach will ensure a high-performing application that can adapt to growth and maintain user satisfaction.
Architecture for Scalability
Designing a scalable booking application for high traffic requires a robust and adaptable architecture. A microservices approach, combined with a well-chosen database and efficient load balancing, is crucial for handling peak demand and ensuring consistent performance. This section details the architectural choices necessary to achieve this scalability.
Microservices Architecture Design
We will employ a microservices architecture, breaking down the application into smaller, independent services. This approach allows for individual scaling of specific components based on their needs, improving resource utilization and fault isolation. Each service will have its own database and can be deployed and updated independently.
- Booking Service: Handles the core booking logic, including availability checks, reservation creation, and modification.
- Payment Service: Processes payments through various gateways, ensuring secure transactions.
- User Service: Manages user accounts, profiles, and authentication.
- Inventory Service: Manages the availability of resources (e.g., hotel rooms, flight seats).
- Notification Service: Sends booking confirmations, reminders, and updates to users via email or SMS.
These services will communicate via asynchronous messaging (e.g., using message queues like Kafka or RabbitMQ) to minimize coupling and improve resilience. This allows services to operate independently and scale individually without impacting the entire system.
Database Selection, Building a scalable booking application for high traffic
Choosing the right database is crucial for scalability. Relational databases (RDBMS) offer strong data consistency and ACID properties, while NoSQL databases excel at handling large volumes of unstructured or semi-structured data and offer horizontal scalability. The choice depends on the specific needs of each microservice. For example, the user service might benefit from a relational database for its structured data, while the inventory service might use a NoSQL database for its potentially massive and rapidly changing data.
Database Type | Scalability | Cost | Ease of Integration |
---|---|---|---|
PostgreSQL (Relational) | Vertically scalable, can be clustered for horizontal scalability but more complex | Moderate to High (depending on usage and infrastructure) | Relatively easy with established tools and libraries |
MySQL (Relational) | Similar to PostgreSQL, good for read-heavy workloads with master-slave replication | Moderate (generally less expensive than PostgreSQL) | Easy to integrate with most programming languages |
MongoDB (NoSQL – Document) | Highly scalable horizontally, easily handles large datasets | Moderate to High (depending on usage and cloud provider) | Easy integration with many languages and frameworks |
Cassandra (NoSQL – Wide-column store) | Excellent horizontal scalability, designed for high availability and fault tolerance | Moderate to High (depending on cluster size and cloud provider) | More complex integration compared to MongoDB, requires understanding of its data model |
Load Balancing Strategy
To distribute traffic evenly across multiple servers, a load balancer will be implemented. A reverse proxy load balancer, such as Nginx or HAProxy, will sit in front of the application servers. This load balancer will distribute incoming requests based on various algorithms (round-robin, least connections, etc.), ensuring that no single server is overloaded. This also provides a single point of entry for clients, abstracting away the complexity of the backend infrastructure.
Additionally, a content delivery network (CDN) can be used to cache static content closer to users, reducing the load on the application servers.
System Architecture Diagram
[Imagine a diagram here. The diagram would show clients connecting to a reverse proxy load balancer (e.g., Nginx). The load balancer distributes traffic to multiple instances of each microservice (Booking, Payment, User, Inventory, Notification). Each microservice has its own database (relational or NoSQL, as appropriate). Asynchronous messaging (e.g., Kafka) is shown connecting the microservices.
A CDN sits in front of the load balancer to cache static assets.] This architecture ensures high availability and scalability by distributing the load across multiple servers and enabling independent scaling of individual microservices. The use of asynchronous messaging decouples the services, improving resilience and allowing for independent deployments and updates. The CDN further improves performance by serving static content from geographically distributed servers.
Handling High Concurrency
Building a scalable booking application requires robust strategies for managing a high volume of concurrent requests. Failure to do so can lead to slow response times, service outages, and ultimately, lost revenue. This section details techniques to ensure your application remains responsive and reliable even under peak loads.
Efficiently handling concurrent requests involves a multifaceted approach, combining architectural choices, optimized database interactions, and sophisticated queueing systems. The key is to distribute the workload effectively, prevent data corruption, and maintain a consistent user experience regardless of the traffic volume.
Queuing Systems and Asynchronous Processing
Implementing a message queue, such as RabbitMQ or Kafka, allows the application to decouple request processing from immediate response. Instead of directly handling each request synchronously, the application adds it to a queue. Separate worker processes then consume these requests and process them asynchronously. This prevents the application server from being overwhelmed by a sudden surge in requests.
For example, imagine a booking system where processing a reservation involves verifying availability, updating inventory, and sending confirmation emails. With asynchronous processing, the user receives an immediate acknowledgement that their request is being processed, while the background workers handle the more time-consuming tasks. This improves user experience and allows the system to handle more requests concurrently.
Preventing Race Conditions and Data Inconsistencies
Race conditions occur when multiple processes access and modify shared data simultaneously, leading to unpredictable results. To mitigate this, several techniques are crucial. Optimistic locking uses version numbers or timestamps to detect conflicts. Before updating data, the system checks if the version number matches the expected value. If not, it indicates a conflict, and the operation is retried.
Pessimistic locking, on the other hand, acquires exclusive locks on data before accessing it, preventing concurrent modifications. However, pessimistic locking can significantly reduce concurrency. Transactions provide an atomic unit of work, ensuring that either all operations within a transaction succeed, or none do. This guarantees data consistency even in the face of concurrent requests. For example, consider updating the availability of a flight seat.
A transaction would ensure that the seat is only marked as booked after successfully updating the database and sending the confirmation email. Failure at any stage would rollback the entire transaction, maintaining data integrity.
Optimizing Database Queries
Database performance is critical under heavy load. Indexing crucial columns allows the database to quickly locate relevant data. Properly designed indexes can drastically reduce query execution time. Using parameterized queries or prepared statements prevents SQL injection vulnerabilities and improves performance by reusing compiled query plans. Caching frequently accessed data, either in-memory or using a distributed cache like Redis, further minimizes database load.
For instance, caching popular flight routes or hotel availability significantly reduces the number of database queries required to serve user requests. Additionally, database connection pooling helps to efficiently manage database connections, minimizing the overhead of establishing new connections for each request.
Handling Traffic Spikes
Unexpected traffic spikes can overwhelm even well-designed systems. Horizontal scaling, adding more application servers to distribute the load, is a key strategy. Load balancers distribute incoming requests across multiple servers, preventing any single server from being overloaded. Auto-scaling features in cloud environments automatically adjust the number of servers based on current demand. Furthermore, techniques like rate limiting can help control the rate of incoming requests, preventing the system from being completely overwhelmed.
For example, a surge of bookings during a flash sale could be mitigated by implementing rate limiting to restrict the number of requests per user or per IP address within a given time frame. This protects the system from being flooded and allows for a more controlled processing of requests.
Caching Strategies
Effective caching is paramount for a high-traffic booking application. By strategically storing frequently accessed data closer to the user, we can significantly reduce database load, improve response times, and enhance the overall user experience. This section details various caching mechanisms and their implementation within our system.
Caching Mechanisms and Their Applications
Our booking system will leverage a multi-level caching architecture, incorporating several mechanisms to optimize performance. Content Delivery Networks (CDNs) will cache static assets like images and CSS files, reducing server load and improving latency for geographically dispersed users. An in-memory cache, such as Redis, will store frequently accessed dynamic data, providing extremely fast read access. Finally, database caching will optimize queries to frequently accessed database tables.
Caching Strategy Comparison for Different Data Types
Different data types necessitate different caching strategies. The product catalog, relatively static, benefits greatly from CDN and in-memory caching. Updates are infrequent, so cache invalidation is less critical. User data, frequently updated, requires a more dynamic approach. In-memory caching is crucial for fast retrieval of user profiles and preferences.
However, a robust cache invalidation strategy is needed to ensure data consistency. Booking information, highly dynamic and transactional, demands careful consideration. While in-memory caching can speed up read operations, write-through caching with eventual consistency might be necessary to balance speed and data accuracy. Database caching can be beneficial for frequently accessed booking details, such as availability and pricing.
Building a scalable booking application for high traffic necessitates robust architecture and efficient resource management. Consider, for example, how integrating features like automated check-in could improve user experience, a concept similar to the convenience offered by smart home technology integration for stylish interior design. Ultimately, seamless user journeys are key to success in a high-traffic booking system, mirroring the ease of use expected in a modern smart home.
Multi-Level Caching System Implementation Plan
Our multi-level caching system will integrate CDN, in-memory cache (Redis), and database caching. The CDN will serve static assets. Redis will cache frequently accessed data such as product details, user profiles (with appropriate privacy considerations), and recently viewed bookings. Database caching will focus on frequently queried tables, such as pricing and availability. The system will prioritize in-memory cache hits, falling back to the database only when necessary.
This layered approach ensures optimal performance and scalability. For example, a user viewing a product page will first check the CDN for images and CSS. Then, Redis will be checked for product details. If not found, the database will be queried, and the result will be stored in Redis for future requests.
Cache Invalidation Strategy
Maintaining data consistency is crucial. Our cache invalidation strategy will employ a combination of techniques. For the CDN, a content versioning system will ensure that updated assets are correctly distributed. For Redis, we will use a combination of time-to-live (TTL) settings and publish/subscribe messaging to invalidate entries when data changes in the database. Database caching will leverage database triggers or change data capture (CDC) mechanisms to automatically invalidate cached queries upon data modification.
This proactive approach minimizes stale data and ensures data consistency across all cache layers. For example, if a booking is made, the system will publish a message to Redis, invalidating the cached availability information. This message will trigger updates in Redis, ensuring that subsequent requests reflect the updated booking status.
API Design and Optimization
A well-designed and optimized API is crucial for a scalable booking application, ensuring efficient communication between the application’s various components and external systems. This section details the API design, potential bottlenecks, optimization strategies, rate limiting, and versioning techniques.
Our RESTful API will adhere to standard HTTP methods and predictable resource URLs, focusing on simplicity and ease of integration for both internal and external clients. This approach prioritizes clarity and maintainability, contributing to the overall scalability and robustness of the system.
RESTful API Endpoints and Data Structures
The API will utilize standard HTTP methods (GET, POST, PUT, DELETE) to manage booking resources. Endpoints will be designed using a consistent and intuitive structure, allowing for easy navigation and understanding. For example, `/bookings` will handle listing, creating, updating, and deleting bookings. Data structures will be represented using JSON, providing a lightweight and easily parsable format. Specific endpoints might include `/bookings/bookingId`, `/users/userId/bookings`, and `/hotels/hotelId/availability`.
The data structures for each endpoint will be carefully defined to include only necessary fields, minimizing data transfer overhead. For example, a booking object might contain fields like `bookingId`, `userId`, `hotelId`, `checkInDate`, `checkOutDate`, `numberOfGuests`, and `totalPrice`.
Potential API Bottlenecks and Optimization Solutions
Several potential bottlenecks can impact API performance. Database queries are a primary concern; inefficient queries can lead to significant delays. Solutions include optimizing database schema, using appropriate indexing strategies, and employing connection pooling to manage database connections effectively. Another potential bottleneck is the serialization and deserialization of JSON data. Using efficient libraries and minimizing unnecessary data transfer can significantly improve performance.
Caching frequently accessed data using technologies like Redis can also dramatically reduce database load. Load balancing across multiple API servers ensures that no single server becomes overloaded. Asynchronous processing of tasks, such as sending email confirmations, helps prevent blocking operations and improves overall responsiveness.
API Rate Limiting and Throttling
Implementing rate limiting and throttling mechanisms is vital for preventing abuse and ensuring system stability. Rate limiting restricts the number of requests a client can make within a specific time window, while throttling slows down the rate of requests when limits are approached. This can be implemented using a combination of techniques, including token buckets, leaky buckets, and sliding windows.
These mechanisms can be configured based on different factors, such as user roles, IP addresses, or API keys, to allow for more flexibility and customization. For example, a free user might have a lower request limit than a premium user. The system should also provide clear error messages when rate limits are exceeded.
API Versioning Strategies
API versioning is crucial for accommodating future changes and maintaining backward compatibility. We will utilize URI versioning, incorporating the version number directly into the URL (e.g., `/v1/bookings`). This allows for multiple API versions to coexist, enabling gradual updates and minimizing disruption to existing clients. A clear deprecation policy will be implemented, providing ample notice to clients before older versions are retired.
This approach provides a clear path for upgrades and ensures a smooth transition for clients using older versions of the API. Comprehensive documentation for each API version will be maintained to facilitate seamless integration and updates.
Testing and Monitoring
A robust testing and monitoring strategy is crucial for a high-traffic booking application. Thorough testing ensures the application functions correctly under various conditions, while comprehensive monitoring allows for proactive identification and resolution of performance bottlenecks. This proactive approach minimizes downtime and maintains a positive user experience.A multi-layered approach to testing is essential, encompassing unit, integration, and performance testing.
Monitoring should include real-time dashboards, alerts, and log analysis to provide a comprehensive view of the application’s health and performance.
Building a scalable booking application for high traffic requires careful consideration of architecture and resource allocation. Think about the user experience; even seemingly small details matter. For instance, a well-designed interface can significantly improve user satisfaction, much like choosing the right smart home lighting solutions for enhancing interior ambiance can transform a space. Returning to our booking app, robust database design is key to handling the expected volume of requests and ensuring smooth operation under pressure.
Unit Testing
Unit tests focus on individual components or modules of the application. These tests verify that each component functions correctly in isolation. For example, a unit test might check that a specific function correctly calculates the total price of a booking, given various inputs. Effective unit testing improves code quality, reduces integration issues, and simplifies debugging. A high unit test coverage, ideally aiming for 80% or higher, is highly recommended.
Popular unit testing frameworks include JUnit (Java), pytest (Python), and Jest (JavaScript).
Building a scalable booking application for high traffic demands robust architecture and efficient resource management. This parallels the need for considered planning when achieving a sustainable and smart home interior design , where thoughtful choices optimize space and resources. Similarly, foresight in application design prevents bottlenecks and ensures a smooth user experience, even under peak demand.
Integration Testing
Integration testing verifies the interaction between different components of the application. This ensures that components work correctly together, even when handling complex booking scenarios involving multiple services (payment gateway, email notifications, etc.). Integration tests often involve mocking or stubbing external dependencies to isolate the interaction between internal components. For instance, an integration test might simulate a payment gateway failure to verify that the application handles the error gracefully.
Performance Testing
Performance testing evaluates the application’s responsiveness and stability under various load conditions. This involves simulating realistic user traffic to identify potential bottlenecks and performance issues. This is critical for a high-traffic booking application. Load testing tools such as JMeter, Gatling, and k6 can simulate a large number of concurrent users, measuring response times, throughput, and resource utilization.
Performance testing helps to identify areas for optimization, ensuring the application can handle peak demand without performance degradation. Key performance indicators (KPIs) to monitor include response time, throughput, error rate, and resource utilization (CPU, memory, network).
Performance Monitoring Tools and Metrics
Several tools are available to monitor the application’s performance in real-time. Application Performance Monitoring (APM) tools like Datadog, New Relic, and Dynatrace provide comprehensive insights into application behavior, including response times, error rates, and resource usage. These tools often integrate with cloud platforms like AWS and Azure, providing centralized monitoring and alerting capabilities. Key metrics to monitor include average response time, 95th percentile response time (to identify outliers), throughput (requests per second), error rate, and resource utilization (CPU, memory, network I/O).
Alerting and Monitoring Dashboards
Setting up alerts and monitoring dashboards is critical for proactive issue identification. Monitoring dashboards should provide a real-time view of key performance indicators, allowing developers to quickly identify and address performance issues. Alerts should be configured to notify the development team when critical metrics exceed predefined thresholds (e.g., high error rates, slow response times, high CPU utilization). These alerts can be integrated with communication channels such as email, Slack, or PagerDuty.
A well-designed dashboard provides a clear overview of the application’s health, enabling swift responses to potential problems.
Rollback Plan
A robust rollback plan is essential to mitigate the impact of unexpected issues or performance degradation. This plan should detail the steps required to revert to a previously stable version of the application. This may involve deploying a previous version of the code from version control, restoring data from backups, and potentially scaling down resources to manage the load until the issue is resolved.
Regular backups of the application code and database are crucial for a successful rollback. The rollback process should be well-documented and tested regularly to ensure its effectiveness in a crisis.
Security Considerations
Building a scalable booking application requires robust security measures to protect sensitive user data and maintain the trust of customers. A breach can have devastating consequences, impacting reputation, finances, and legal compliance. Therefore, a comprehensive security strategy must be integrated into every stage of development and deployment.
Protecting user data and preventing unauthorized access necessitates a multi-layered approach encompassing various security best practices. This involves not only technical solutions but also robust security policies and employee training programs. The high-traffic nature of the application further emphasizes the need for proactive security measures to withstand potential attacks.
Data Protection and Access Control
Protecting sensitive user data, such as payment information, personal details, and travel itineraries, is paramount. Data encryption both in transit (using HTTPS) and at rest (using database encryption) is essential. Access control mechanisms, such as role-based access control (RBAC), should be implemented to restrict access to sensitive data based on user roles and responsibilities. Regular security audits and penetration testing are crucial to identify and address vulnerabilities.
Building a scalable booking application for high traffic demands careful planning and robust infrastructure. The developer’s workspace significantly impacts productivity; consider investing time in designing a smart home office with ergonomic and stylish furniture to optimize performance. A comfortable and efficient workspace directly contributes to the success of such a demanding project, ultimately leading to a more effective development process for your high-traffic booking application.
Data loss prevention (DLP) measures should be in place to prevent sensitive data from leaving the system’s controlled environment. Compliance with relevant data privacy regulations, such as GDPR and CCPA, is mandatory.
Potential Security Vulnerabilities and Mitigation Strategies
High-traffic booking applications are attractive targets for various attacks. SQL injection vulnerabilities can be mitigated through parameterized queries and input validation. Cross-site scripting (XSS) attacks can be prevented by proper input sanitization and output encoding. Cross-site request forgery (CSRF) attacks can be mitigated using CSRF tokens. Denial-of-service (DoS) attacks can be mitigated through robust infrastructure design, load balancing, and rate limiting.
Regular security assessments and penetration testing, performed by both internal and external security experts, are crucial for identifying and addressing vulnerabilities proactively. Employing a web application firewall (WAF) can provide an additional layer of protection against common web attacks.
Secure Authentication and Authorization
Implementing strong authentication mechanisms is crucial. Multi-factor authentication (MFA) should be strongly considered, requiring users to provide multiple forms of authentication, such as passwords, one-time codes, and biometric data. Password management should follow best practices, including strong password policies and password hashing using secure algorithms like bcrypt or Argon2. Authorization mechanisms, such as OAuth 2.0 or OpenID Connect, should be used to control access to specific resources based on user roles and permissions.
Session management should be secure, using short-lived sessions and secure cookies with appropriate flags (e.g., HttpOnly, Secure).
Building a scalable booking application for high traffic necessitates robust infrastructure and efficient resource management. Consider the user experience; for instance, imagine the seamless integration of smart home controls, such as those detailed in this article on smart home automation for improved interior comfort and convenience , to enhance the pre-arrival experience for guests. This level of user-centric design directly impacts the overall scalability and success of the booking application.
Security Incident Handling and Response
A well-defined incident response plan is crucial for handling security incidents effectively. This plan should Artikel procedures for identifying, containing, eradicating, recovering from, and learning from security incidents. A dedicated security team should be responsible for monitoring systems, investigating incidents, and coordinating responses. Regular security awareness training for employees is essential to prevent human error from becoming a security vulnerability.
The plan should include communication protocols for notifying affected users and regulatory bodies as needed. Post-incident analysis should be conducted to identify root causes and implement preventative measures to avoid future incidents. This includes logging and monitoring of all security-relevant events for later analysis and auditing purposes.
Deployment and Scaling
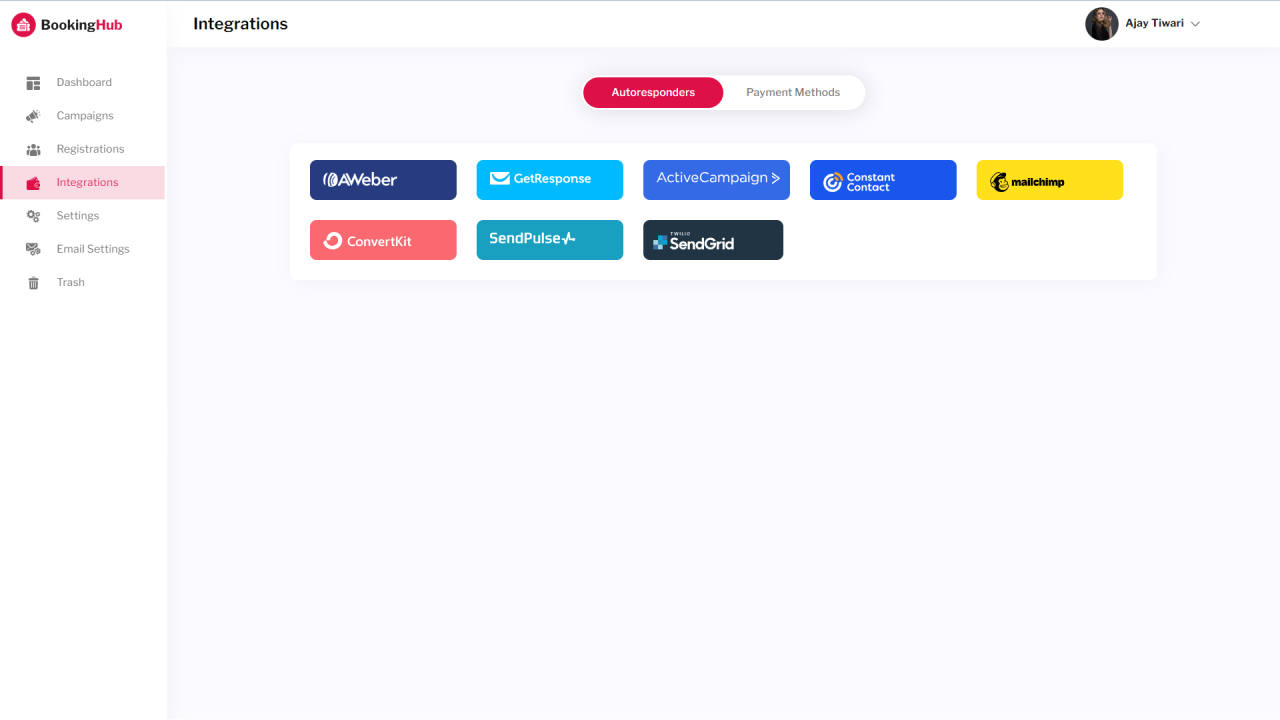
Source: envato.com
Designing a scalable booking application for high-volume traffic necessitates careful consideration of database architecture and server infrastructure. However, even developers need a break! For inspiration, check out these best smart home interior design ideas for small apartments to create a relaxing space away from the screen. Returning to the application, efficient caching strategies are crucial for maintaining responsiveness under peak loads.
Deploying a high-traffic booking application requires a robust and scalable strategy. This involves careful consideration of infrastructure, deployment methodologies, and scaling techniques to ensure high availability, performance, and cost-effectiveness. The chosen approach must allow for seamless updates and efficient resource utilization, adapting to fluctuating user demand.Deployment Strategy for Scalability and ResilienceA blue/green deployment strategy offers a high degree of resilience and minimizes downtime.
In this approach, two identical environments (blue and green) exist. New code is deployed to the inactive environment (e.g., green), thoroughly tested, and then traffic is switched from the active (blue) to the new (green) environment. If issues arise, traffic can be quickly switched back to the blue environment. This minimizes disruption to users and allows for rapid rollback if necessary.
Automated testing and continuous integration/continuous delivery (CI/CD) pipelines are crucial for efficient and reliable deployments within this model.
Horizontal and Vertical Scaling
Horizontal scaling involves adding more servers to the application pool to handle increased load. This distributes the workload across multiple machines, preventing any single server from becoming overloaded. Vertical scaling, on the other hand, involves increasing the resources (CPU, RAM, etc.) of existing servers. While simpler to implement initially, vertical scaling has limitations as there’s a practical upper bound to the resources a single server can handle.
A hybrid approach, combining both horizontal and vertical scaling, is often the most effective strategy for managing fluctuating demand. For example, during peak booking periods, horizontal scaling can be triggered to add more servers, while vertical scaling might be used to upgrade existing servers to handle increased traffic in the off-peak period.
Containerization and Orchestration
Containerization, using tools like Docker, packages the application and its dependencies into isolated units, ensuring consistent execution across different environments. Orchestration tools, such as Kubernetes, manage the deployment, scaling, and networking of these containers across a cluster of servers. Kubernetes automates many of the complex tasks involved in managing a distributed system, including load balancing, service discovery, and rolling updates.
This simplifies deployment and allows for efficient scaling of the application in response to changing demand. Using Kubernetes, for example, we can define deployments that automatically scale up or down based on CPU utilization or other metrics. This ensures that resources are allocated efficiently and costs are optimized.
Infrastructure Cost Management
Managing infrastructure costs while maintaining performance and scalability requires a multi-faceted approach. Leveraging cloud-based services with auto-scaling capabilities allows for dynamic resource allocation, paying only for what’s used. Right-sizing instances, choosing the appropriate server sizes based on predicted load, is crucial. Employing cost optimization tools provided by cloud providers helps monitor resource utilization and identify areas for improvement.
Furthermore, utilizing spot instances (preemptible VMs) can significantly reduce costs, although this approach requires careful consideration of application resilience as these instances can be terminated with short notice. For example, Amazon Web Services offers a range of cost optimization tools and pricing models, allowing for flexible management of infrastructure expenses. By strategically utilizing these options and continuously monitoring resource consumption, we can minimize costs without compromising performance or scalability.
Final Review: Building A Scalable Booking Application For High Traffic
Creating a scalable booking application capable of handling high traffic demands a holistic approach, encompassing architectural design, concurrency management, caching strategies, API optimization, rigorous testing, and robust security measures. By carefully considering each of these elements, developers can build a system that not only meets current needs but also adapts gracefully to future growth and changing user demands. The resulting application will provide a reliable and efficient booking experience, ensuring user satisfaction and business success.
FAQ
What are the key performance indicators (KPIs) to monitor in a high-traffic booking application?
Key KPIs include response times, error rates, request throughput, database query latency, and resource utilization (CPU, memory, network). Monitoring these metrics provides insights into application performance and helps identify potential bottlenecks.
How can I prevent denial-of-service (DoS) attacks on my booking application?
Implementing measures such as rate limiting, input validation, and using a web application firewall (WAF) are crucial in mitigating DoS attacks. Distributed denial-of-service (DDoS) protection often requires employing a cloud-based DDoS mitigation service.
What are some strategies for handling database migrations in a live, high-traffic environment?
Employ blue-green deployments or canary releases to minimize downtime during database migrations. These techniques allow for gradual rollout and rollback capabilities, ensuring minimal disruption to users.
How do I choose the right cloud provider for my scalable booking application?
Consider factors like cost, scalability options, geographic reach, security features, and the provider’s support for your chosen technologies (e.g., container orchestration, databases). Evaluate offerings from multiple providers to find the best fit for your specific needs.
- High-performance glass A detailed look - June 2, 2025
- Coastal Modern House A Guide - May 6, 2025
- Floral Centerpieces A Complete Guide - April 20, 2025