How to build a robust booking application architecture is a multifaceted challenge demanding careful consideration of several key areas. Successfully navigating database design, API implementation, scalability, user experience, and security is crucial for creating a reliable and user-friendly system. This guide provides a comprehensive overview of the architectural considerations necessary to build a high-performing and secure booking application.
From defining a robust database schema to implementing secure payment gateways and employing effective scaling strategies, each stage of development requires careful planning and execution. We’ll explore the intricacies of RESTful API design, the importance of user-centric UI/UX, and the critical role of comprehensive testing and deployment procedures. Understanding these elements is vital for building a booking application that can handle significant user traffic and maintain data integrity.
Database Design for a Booking Application
Designing a robust database schema is crucial for a successful booking application. A well-structured database ensures data integrity, efficient querying, and scalability as the application grows. This section details the design of a relational database for a booking application, covering schema design, normalization, and database technology selection.
Relational Database Schema
The core components of our booking application database are users, bookings, resources, and payments. These entities are interconnected, forming a relational model. The following ER diagram illustrates these relationships:(Imagine an ER diagram here. It would show four entities: Users, Bookings, Resources, and Payments. Users would have attributes like user_id (PK), name, email, password.
Bookings would have booking_id (PK), user_id (FK referencing Users), resource_id (FK referencing Resources), booking_date, booking_time, etc. Resources would have resource_id (PK), resource_type (e.g., ‘room’, ‘equipment’), name, description. Payments would have payment_id (PK), booking_id (FK referencing Bookings), payment_method, amount, payment_date. Relationships would be clearly indicated, showing the one-to-many relationships between Users and Bookings, Resources and Bookings, and Bookings and Payments.)The following table Artikels the database schema:
Table Name | Column Name | Data Type | Constraints | Relationship |
---|---|---|---|---|
Users | user_id | INT | PRIMARY KEY, AUTO_INCREMENT | |
Users | name | VARCHAR(255) | NOT NULL | |
Users | VARCHAR(255) | UNIQUE, NOT NULL | ||
Users | password | VARCHAR(255) | NOT NULL | |
Resources | resource_id | INT | PRIMARY KEY, AUTO_INCREMENT | |
Resources | resource_type | VARCHAR(50) | NOT NULL | |
Resources | name | VARCHAR(255) | NOT NULL | |
Resources | description | TEXT | ||
Bookings | booking_id | INT | PRIMARY KEY, AUTO_INCREMENT | |
Bookings | user_id | INT | FOREIGN KEY (Users) | One-to-many with Users |
Bookings | resource_id | INT | FOREIGN KEY (Resources) | One-to-many with Resources |
Bookings | booking_date | DATE | NOT NULL | |
Bookings | booking_time | TIME | NOT NULL | |
Payments | payment_id | INT | PRIMARY KEY, AUTO_INCREMENT | |
Payments | booking_id | INT | FOREIGN KEY (Bookings) | One-to-many with Bookings |
Payments | payment_method | VARCHAR(50) | NOT NULL | |
Payments | amount | DECIMAL(10,2) | NOT NULL | |
Payments | payment_date | TIMESTAMP | NOT NULL |
Database Normalization
The database schema is designed using the principles of database normalization, specifically aiming for at least the third normal form (3NF). This involves eliminating data redundancy and improving data integrity. For example, user information is stored only once in the `Users` table, and bookings reference users via the `user_id` foreign key. This prevents inconsistencies and simplifies data updates. Further normalization could be considered depending on the complexity of the application and the potential for future data expansion.
Database Technology Selection
PostgreSQL is chosen as the database technology. PostgreSQL is a powerful, open-source, relational database system known for its robustness, scalability, and support for advanced features like JSONB for storing complex data. Its ACID properties guarantee data consistency and reliability, which is crucial for a booking application where accurate and consistent data is paramount. Other options like MySQL are viable, but PostgreSQL’s features and community support make it a more suitable choice for a system that may need to handle a large volume of bookings and complex queries.
API Design and Implementation
A well-designed API is crucial for a robust booking application. It acts as the interface between the application’s frontend and backend, enabling seamless communication and data exchange. A RESTful approach, leveraging standard HTTP methods and JSON data format, is ideal for its simplicity, scalability, and wide adoption. This section details the design and implementation of the APIs for our booking application, focusing on user management, resource management, and booking management.
RESTful API Design for Booking Application
The API will utilize a RESTful architecture, employing standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. Resources are identified by URLs, and each method performs a specific action. The responses are formatted in JSON for easy parsing and consumption by various clients (web, mobile, etc.). We will employ consistent naming conventions for endpoints and data structures to enhance readability and maintainability.
Below are examples of key API endpoints.
API Endpoints
Endpoint | HTTP Method | Request Parameters | Response Codes | Request Body (Example) | Response Body (Example) |
---|---|---|---|---|---|
/users | POST | None | 201 (Created), 400 (Bad Request) | "username": "john.doe", "email": "john.doe@example.com", "password": "securepassword" |
"id": 1, "username": "john.doe", "email": "john.doe@example.com" |
/users/userId | GET | userId | 200 (OK), 404 (Not Found) | None | "id": 1, "username": "john.doe", "email": "john.doe@example.com" |
/resources | GET | None | 200 (OK) | None | ["id": 1, "name": "Conference Room A", "capacity": 10, "id": 2, "name": "Meeting Room B", "capacity": 5] |
/bookings | POST | None | 201 (Created), 400 (Bad Request), 409 (Conflict) | "resourceId": 1, "userId": 1, "startTime": "2024-03-15T10:00:00", "endTime": "2024-03-15T11:00:00" |
"id": 1, "resourceId": 1, "userId": 1, "startTime": "2024-03-15T10:00:00", "endTime": "2024-03-15T11:00:00" |
/bookings/bookingId | DELETE | bookingId | 204 (No Content), 404 (Not Found) | None | None |
API Authentication and Authorization
The API will implement OAuth 2.0 for authentication and authorization. OAuth 2.0 allows third-party applications to access user resources without sharing the user’s credentials. This enhances security and allows for granular control over access permissions. The process involves obtaining an access token through an authorization server, which is then included in subsequent API requests as a Bearer token in the Authorization header.
This token verifies the user’s identity and grants access to specific resources based on their roles and permissions. JWT (JSON Web Tokens) could be used as the access token format due to its compact and self-contained nature.
Scalability and Performance: How To Build A Robust Booking Application Architecture
Building a robust booking application requires careful consideration of scalability and performance to ensure a smooth user experience even under heavy load. A system that performs well with a few users might crumble under the weight of thousands of concurrent bookings. This section Artikels strategies to address these challenges.
A key aspect of building a scalable and performant booking application is anticipating potential bottlenecks and implementing proactive solutions. This includes careful database design, efficient API implementation, and strategic use of caching and load balancing.
Designing a robust booking application architecture requires careful consideration of scalability and security. For instance, ensuring user data protection is paramount, much like the need for robust security in a smart home; consider exploring options like smart home security systems that complement interior aesthetics for inspiration on integrated security measures. Returning to the booking app, a well-structured database and efficient API are crucial for a seamless user experience.
Load Balancing
Load balancing distributes incoming traffic across multiple servers, preventing any single server from becoming overloaded. This is crucial for handling peak demand periods, such as holiday seasons or special events. A common approach is to use a load balancer that acts as a reverse proxy, directing requests to available servers based on factors like server load and health.
For example, a round-robin algorithm distributes requests evenly, while a more sophisticated algorithm might consider server performance metrics to optimize distribution. This ensures that no single server is overwhelmed, maintaining consistent response times and preventing service disruptions.
Caching
Caching stores frequently accessed data in a readily available location, reducing the need to repeatedly fetch it from slower storage like databases. This significantly improves response times. Various caching strategies exist, including:
- Database caching: Caching frequently queried data within the database itself using techniques like query caching.
- Application-level caching: Storing frequently accessed data in memory (e.g., using Redis or Memcached) within the application servers. This can cache booking availability, user profiles, or other frequently used data.
- CDN caching: Utilizing a Content Delivery Network (CDN) to cache static assets (images, CSS, JavaScript) closer to users, reducing latency.
Effective caching requires careful consideration of cache invalidation strategies to ensure data consistency.
Database Sharding
Database sharding horizontally partitions a large database across multiple smaller databases. This distributes the data load, improving scalability and performance. For a booking application, sharding might involve partitioning data based on geographical location, user ID, or booking date. This approach allows for parallel processing of queries and prevents a single database server from becoming a bottleneck. However, careful planning is needed to manage data consistency and query complexity across shards.
Potential Performance Bottlenecks and Solutions
Several areas within a booking application are prone to performance bottlenecks.
- Database queries: Inefficiently written database queries can significantly impact performance. Solutions include optimizing queries using appropriate indexes, using stored procedures, and avoiding unnecessary joins.
- API endpoints: Slow API responses can hinder the user experience. Solutions involve optimizing API code, using asynchronous processing, and implementing caching strategies.
- Third-party integrations: Reliance on external services can introduce latency. Solutions include choosing reliable and performant third-party services and implementing robust error handling.
- Lack of sufficient resources: Insufficient server resources (CPU, memory, network bandwidth) can lead to performance degradation. Solutions include scaling up server resources (vertical scaling) or adding more servers (horizontal scaling).
Database Query Optimization
Optimizing database queries is critical for application performance. Strategies include:
- Indexing: Creating indexes on frequently queried columns significantly speeds up data retrieval. For example, an index on the `booking_date` column would greatly improve the speed of queries searching for bookings on a specific date.
- Query optimization techniques: Using techniques like `EXPLAIN` (or equivalent in your database system) to analyze query execution plans and identify areas for improvement. This allows for identifying slow queries and optimizing their performance.
- Stored procedures: Using stored procedures can improve performance by pre-compiling and optimizing database queries.
- Database connection pooling: Reusing database connections instead of creating new ones for each request reduces overhead and improves performance.
User Interface (UI) and User Experience (UX) Considerations
A robust booking application requires a user-friendly interface and a seamless user experience to ensure high user adoption and satisfaction. The design should prioritize ease of navigation, clear information presentation, and efficient task completion. A well-designed UI/UX fosters trust and encourages repeat bookings.A well-designed UI/UX is crucial for a successful booking application. It should be intuitive, visually appealing, and efficient, allowing users to easily find, book, and manage their reservations.
A poor UI/UX can lead to frustration, abandoned bookings, and ultimately, lost revenue.
User Interface Design
The user interface should be clean, uncluttered, and visually appealing. A consistent design language with clear visual hierarchy is essential for guiding users through the booking process. The main screen should feature a prominent search bar, allowing users to quickly filter available resources by date, time, location, and other relevant criteria. A calendar view integrated with the search results will help users visualize availability.
Once a resource is selected, detailed information such as pricing, description, and photos should be readily accessible. A clear and concise booking form should follow, requiring only essential information from the user. Progress indicators during the booking process will provide users with a sense of control and transparency. A simple and effective design will contribute to a positive user experience and increase conversion rates.
Designing a robust booking application architecture requires careful consideration of scalability and user experience. For instance, integrating features that anticipate user needs, such as automated confirmations, is crucial. This is similar to the proactive nature of smart home automation for improved interior comfort and convenience , where systems anticipate user preferences. Ultimately, a successful booking app, like a well-designed smart home, prioritizes seamless functionality and user satisfaction.
User Experience Flow for Making a Booking
The user experience should be straightforward and intuitive. The booking process typically begins with searching for available resources. The search results should be presented in a clear and organized manner, with relevant filters and sorting options. Users should be able to easily compare different options based on their criteria. Once a suitable resource is selected, the user should be guided through a simple booking form, requesting essential details such as dates, times, and personal information.
Real-time availability checks are critical to prevent double-bookings. Confirmation of the booking should be provided immediately, with a clear summary of the reservation details. Users should also have access to manage their bookings, cancel or modify them if needed, and easily contact customer support if necessary. A seamless and efficient booking process increases user satisfaction and encourages repeat usage.
Responsive Design Implementation
The application must be responsive to ensure it works seamlessly across various devices, including desktops, tablets, and smartphones. This requires using flexible layouts and adapting the UI elements to different screen sizes and resolutions. Responsive design ensures consistent usability and a positive user experience regardless of the device used to access the application. For example, on smaller screens, the UI might condense elements into collapsible menus or utilize swipe gestures for navigation, while maintaining the core functionality.
Thorough testing on different devices and screen sizes is crucial to ensure a consistent experience across all platforms. This approach maximizes user reach and ensures accessibility for a wider audience.
Designing a robust booking application architecture requires careful consideration of scalability and user experience. For instance, think about the level of detail needed; imagine integrating features inspired by the sleek functionality showcased in modern smart home interior design with minimalist furniture , applying that same principle of clean design to your app’s interface. This focus on efficiency and intuitive navigation is crucial for a successful booking system.
Security Considerations
Building a robust booking application requires a proactive approach to security, encompassing all stages from design to deployment. Neglecting security can lead to significant financial losses, reputational damage, and legal repercussions. This section Artikels key security considerations and mitigation strategies for a secure booking system.
Input Validation and Output Encoding
Input validation is crucial for preventing malicious code injection attacks, such as SQL injection and cross-site scripting (XSS). All user inputs, including dates, times, names, and payment details, must be rigorously validated against predefined formats and constraints before being processed by the application. This validation should occur on both the client-side (using JavaScript) and the server-side to prevent circumvention.
Building a robust booking application architecture requires careful consideration of scalability and user experience. A well-designed system should seamlessly handle high volumes of requests, and creating a productive workspace is key; this includes aspects like designing a smart home office with ergonomic and stylish furniture , which can improve focus and efficiency for developers working on the application.
Ultimately, a successful booking app relies on both efficient backend design and a supportive, comfortable development environment.
Output encoding ensures that data displayed to the user is rendered safely, preventing XSS attacks. Special characters should be escaped or encoded before being displayed in HTML, preventing the execution of malicious scripts. For example, user-supplied data displayed on a webpage should be HTML-encoded before rendering. This prevents an attacker from injecting malicious JavaScript code that could steal user data or redirect users to malicious websites.
Secure Coding Practices
Secure coding practices are essential for preventing a wide range of vulnerabilities. Developers should follow secure coding guidelines, such as those provided by OWASP (Open Web Application Security Project), to minimize risks. This includes using parameterized queries to prevent SQL injection, avoiding hard-coded credentials, and implementing proper error handling to prevent information leakage. Regularly updating software libraries and frameworks is crucial to patch known vulnerabilities.
Employing a secure development lifecycle (SDLC) that incorporates security testing at each stage is highly recommended. This might include static and dynamic application security testing (SAST and DAST) to identify vulnerabilities early in the development process.
Secure Payment Processing
Integrating with a reputable payment gateway is paramount for secure payment processing. These gateways handle sensitive payment information, such as credit card numbers and expiry dates, securely, relieving the application of the burden of managing this sensitive data directly. PCI DSS (Payment Card Industry Data Security Standard) compliance is essential when handling credit card information. The application should never store sensitive payment information directly; instead, it should rely on the payment gateway’s tokenization system to represent payment details with secure tokens.
Examples of reputable payment gateways include Stripe, PayPal, and Square. Choosing a gateway with robust security features and a strong track record is vital.
Data Encryption and Access Control, How to build a robust booking application architecture
Protecting user data from unauthorized access requires implementing robust data encryption and access control mechanisms. Sensitive data, such as user credentials, personal information, and booking details, should be encrypted both in transit (using HTTPS) and at rest (using database encryption). Access control mechanisms should restrict access to sensitive data based on user roles and permissions. This might involve implementing role-based access control (RBAC) to limit access to specific functionalities and data based on user roles (e.g., administrator, customer service agent, user).
Regular security audits and penetration testing should be performed to identify and address vulnerabilities. Implementing multi-factor authentication (MFA) adds an extra layer of security by requiring users to provide multiple forms of authentication, such as a password and a one-time code generated by an authenticator app. This makes it significantly harder for attackers to gain unauthorized access even if they obtain a password.
Error Handling and Logging
A robust booking application requires a comprehensive strategy for handling errors and logging events. Effective error handling ensures a positive user experience, even when unexpected issues arise, while detailed logging facilitates debugging, performance monitoring, and security auditing. This section details the design of such a system.Error handling and logging are crucial for maintaining a stable and reliable booking application.
A well-designed system allows for quick identification and resolution of problems, minimizing downtime and improving the overall user experience. It also provides valuable insights into application behavior, enabling proactive improvements and optimizations.
Exception Handling
A multi-layered approach to exception handling is recommended. At the application’s core, specific exceptions should be caught and handled appropriately. For example, a database connection failure should trigger a retry mechanism or display a user-friendly message indicating temporary unavailability. Generic exceptions should be logged and, if possible, converted into more informative messages for the user, avoiding technical jargon.
A final layer of error handling should be implemented at the API gateway level, to catch any unhandled exceptions and return standardized error responses. This prevents exposing internal server errors to the client. This standardized response might include a unique error code and a brief, user-friendly message. For example, a database error could return a code like `DATABASE_ERROR` with a message like “We’re experiencing a temporary issue; please try again later.”
Logging Strategy
A centralized logging system is essential. This system should capture all relevant events, including successful operations, errors, and warnings. The logging system should employ different log levels (e.g., DEBUG, INFO, WARN, ERROR, FATAL) to categorize events based on their severity. Each log entry should include a timestamp, the log level, a unique identifier for the request, the affected component, a concise description of the event, and any relevant contextual information (e.g., user ID, request parameters, error stack trace).Log files should be stored in a structured format, such as JSON, for easier parsing and analysis.
Consider using a dedicated logging server or a cloud-based logging service for scalability and centralized management. Rotation policies should be implemented to manage log file sizes and prevent disk space exhaustion.
Designing a robust booking application architecture requires careful consideration of scalability and user experience. For instance, integrating features that allow users to visualize their bookings, much like the spatial planning offered by top smart home interior design software and apps for planning , could significantly enhance user engagement. Ultimately, a successful booking application prioritizes both efficient backend processes and intuitive front-end interactions.
Log Analysis for Debugging and Monitoring
The logging data can be analyzed using various tools to identify trends, pinpoint performance bottlenecks, and debug errors. For example, analyzing error logs can reveal recurring issues or identify specific components requiring attention. Performance monitoring tools can use log data to track request latency, resource usage, and other key metrics. Correlation of log entries with specific user actions can help identify the root cause of user-reported problems.
For instance, if multiple users report a failure in a specific feature, analysis of logs associated with those users can reveal a pattern, such as a specific database query failing under load. This information can then be used to improve the application’s performance and stability.
Security Considerations in Logging
Sensitive information, such as passwords, credit card details, or personally identifiable information (PII), should never be logged directly. Instead, masked or anonymized versions should be used. Access to log files should be restricted to authorized personnel only. Proper security measures, such as encryption and access control lists, should be implemented to protect log data from unauthorized access or modification.
Regular audits of log access and usage are crucial for ensuring compliance with security policies.
Designing a robust booking application architecture requires careful consideration of scalability and security. Just as planning a luxurious space requires forethought, like when you’re creating a luxurious smart home interior on a budget , you need to prioritize efficient resource allocation. Similarly, a well-structured booking system ensures smooth operations and prevents bottlenecks, leading to a positive user experience.
This careful planning mirrors the approach needed for any complex system.
Testing and Deployment
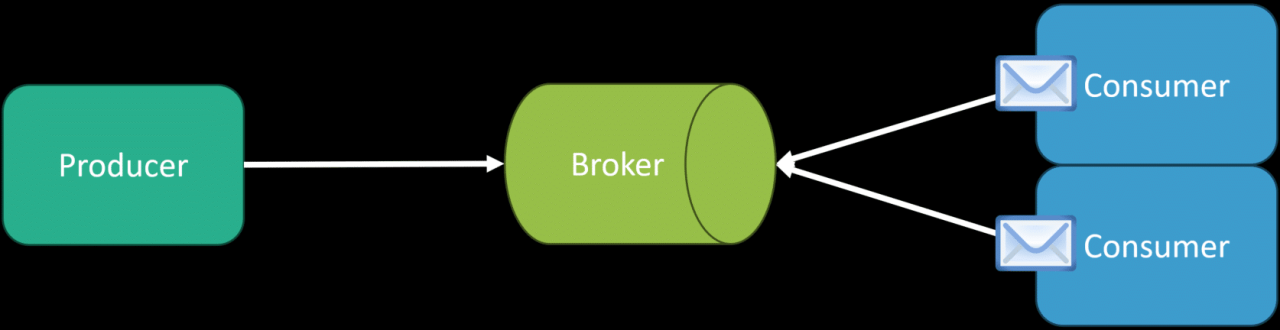
Source: codeopinion.com
A robust testing strategy and a well-defined deployment process are crucial for ensuring the quality, reliability, and scalability of a booking application. Thorough testing identifies and addresses bugs early, preventing costly issues in production. A streamlined deployment process minimizes downtime and ensures smooth updates.
Comprehensive Testing Strategy
A multi-layered testing approach is essential to cover all aspects of the application. This involves unit testing, integration testing, and user acceptance testing (UAT). Unit tests verify individual components, integration tests ensure components work together correctly, and UAT validates the application meets user requirements.
Unit Testing
Unit tests focus on individual units of code, such as functions or classes. These tests should be automated and run frequently as part of the development process. Effective unit tests ensure that each component functions correctly in isolation. For example, a unit test might verify that a function correctly calculates the total price of a booking, considering discounts and taxes.
A comprehensive suite of unit tests will improve code quality and reduce the likelihood of regressions.
Integration Testing
Integration tests verify the interaction between different components of the application. These tests ensure that data flows correctly between modules and that the system behaves as expected as a whole. For example, an integration test might verify that a booking request is correctly processed by the API, the database is updated, and a confirmation email is sent to the user.
This level of testing helps uncover issues related to data consistency and communication between various parts of the system.
User Acceptance Testing (UAT)
UAT involves testing the application with real users to ensure it meets their needs and expectations. This typically involves having users perform real-world tasks within the application, providing feedback on usability, functionality, and overall experience. Feedback from UAT is critical for identifying issues that might not be apparent during earlier testing phases. For instance, UAT might reveal usability problems with the booking interface or unexpected behavior in certain scenarios.
Deployment Process with CI/CD
Continuous Integration and Continuous Deployment (CI/CD) significantly improves the efficiency and reliability of the deployment process. CI involves regularly integrating code changes into a central repository, followed by automated builds and tests. CD automates the deployment process, pushing tested code to production environments. A CI/CD pipeline ensures faster releases, reduced risk, and improved collaboration among developers. A well-structured CI/CD pipeline might include automated unit and integration tests, code quality checks, and deployment to staging environments for final validation before release to production.
Pre-Deployment Checklist
Before deploying any updates, a comprehensive checklist is crucial to prevent unforeseen issues. This checklist should include:
- Verifying that all tests (unit, integration, UAT) have passed successfully.
- Confirming the deployment environment is properly configured and ready.
- Backing up the production database.
- Reviewing the release notes and ensuring all changes are documented.
- Performing a final code review to ensure no critical issues are overlooked.
Post-Deployment Checklist
After deploying the application, several post-deployment tasks are necessary to ensure the successful launch and monitor the system’s health. This includes:
- Monitoring system logs for any errors or unusual behavior.
- Checking key performance indicators (KPIs) such as response times and error rates.
- Gathering user feedback to identify any post-release issues.
- Rolling back to the previous version if necessary, in case of critical failures.
- Documenting the deployment process and any issues encountered.
Final Thoughts
Building a robust booking application architecture requires a holistic approach, encompassing database design, API development, scalability, security, and user experience. By carefully considering each of these elements and implementing best practices, developers can create a system that is not only functional and efficient but also secure and user-friendly. Remember that ongoing monitoring, maintenance, and adaptation are essential to ensure the application’s continued success and resilience in the face of evolving user needs and technological advancements.
Prioritizing a well-defined architecture from the outset significantly contributes to long-term stability and scalability.
FAQ Resource
What are some common database choices for a booking application and their pros and cons?
PostgreSQL offers strong data integrity and scalability, but may have a steeper learning curve. MySQL is widely used, relatively easy to learn, and highly performant for many applications. MongoDB, a NoSQL option, excels with flexibility and scalability but requires careful schema design.
How can I prevent overbooking in my application?
Implement optimistic locking or pessimistic locking mechanisms within your database transactions to ensure that only one booking is made for a specific resource at a given time. This prevents concurrent bookings from clashing.
What are some essential security considerations beyond those mentioned in the Artikel?
Regular security audits, penetration testing, and implementing robust input validation are crucial. Consider using a Web Application Firewall (WAF) to protect against common web attacks. Keep your software and dependencies up-to-date to patch known vulnerabilities.
What are some strategies for handling high traffic during peak booking periods?
Employ load balancing to distribute traffic across multiple servers. Implement caching mechanisms to reduce database load. Consider using a message queue to handle asynchronous tasks and prevent bottlenecks.
- Future Trends and Innovations in Home Security Automation Technology - February 18, 2025
- Creating a Cozy and Inviting Living Room Atmosphere - February 11, 2025
- How to Incorporate Plants into a Modern Living Room - February 11, 2025